|
C语言和C++分别写一套代码,//用递归方法求n阶勒让德多项式的值,递归公式 //pn(x)=1,n=0; pn(x)=x,n=1; pn(X)=((2n-1)*x-pn-1(x)-(n-1)*pn-2(x))/n,n>=1
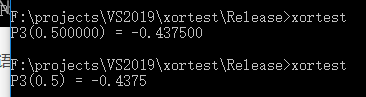
C语言实现:
-
- #include <stdio.h>
- double legendre(int n, double x) {
- if (n == 0) {
- return 1;
- } else if (n == 1) {
- return x;
- } else {
- double pn_2 = 1; // Pn-2(x)
- double pn_1 = x; // Pn-1(x)
- double pn = 0; // Pn(x)
- for (int i = 2; i <= n; i++) {
- pn = ((2 * i - 1) * x * pn_1 - (i - 1) * pn_2) / i;
- pn_2 = pn_1;
- pn_1 = pn;
- }
- return pn;
- }
- }
- int main() {
- int n = 3;
- double x = 0.5;
- double result = legendre(n, x);
- printf("P%d(%f) = %f\n", n, x, result);
- return 0;
- }
复制代码 C++实现:
- #include <iostream>
- double legendre(int n, double x) {
- if (n == 0) {
- return 1;
- } else if (n == 1) {
- return x;
- } else {
- double pn_2 = 1; // Pn-2(x)
- double pn_1 = x; // Pn-1(x)
- double pn = 0; // Pn(x)
- for (int i = 2; i <= n; i++) {
- pn = ((2 * i - 1) * x * pn_1 - (i - 1) * pn_2) / i;
- pn_2 = pn_1;
- pn_1 = pn;
- }
- return pn;
- }
- }
- int main() {
- int n = 3;
- double x = 0.5;
- double result = legendre(n, x);
- std::cout << "P" << n << "(" << x << ") = " << result << std::endl;
- return 0;
- }
复制代码 以上代码分别使用C语言和C++实现了递归方法求解n阶勒让德多项式的值。在主函数中,我们定义了n和x的值,然后调用 legendre 函数计算勒让德多项式的值,并将结果打印输出。
|
|