|
广度优先搜索的原理是:选择一个顶点作为起始点,依次访问该起始点的所有邻接点,再根据邻接点访问他们各自的邻接点,并保证先访问节点的邻接点先与后访问节点的邻接点被访问。
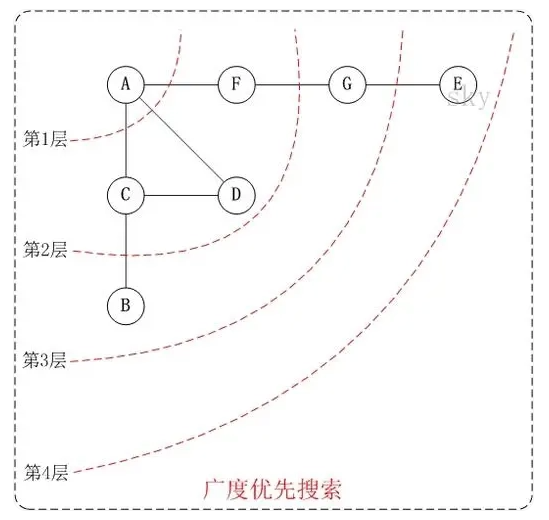
广度搜索算法,分别用C语言,C++,java,python编写出来
当涉及广度优先搜索算法时,下面是使用C语言、C++、Java和Python编写的示例代码:
1.**C语言示例:**
- #include <stdio.h>
- #include <stdbool.h>
- #define MAX_VERTICES 100
- bool visited[MAX_VERTICES];
- int graph[MAX_VERTICES][MAX_VERTICES];
- int numVertices;
- void bfs(int startVertex) {
- bool queue[MAX_VERTICES];
- int front = 0, rear = 0;
- int vertex, i;
- for (i = 0; i < numVertices; i++) {
- visited[i] = false;
- }
- visited[startVertex] = true;
- queue[rear++] = startVertex;
- while (front < rear) {
- vertex = queue[front++];
- printf("%d ", vertex);
- for (i = 0; i < numVertices; i++) {
- if (graph[vertex][i] && !visited[i]) {
- visited[i] = true;
- queue[rear++] = i;
- }
- }
- }
- }
复制代码 int main() {
// Initialize the graph and numVertices
bfs(0);
return 0;
}
2.**C++示例:**
- #include <iostream>
- #include <vector>
- #include <queue>
- using namespace std;
- class Graph {
- int numVertices;
- vector<vector<int>> adjacencyList;
- public:
- Graph(int vertices) {
- numVertices = vertices;
- adjacencyList.resize(numVertices);
- }
- void addEdge(int source, int destination) {
- adjacencyList[source].push_back(destination);
- }
- void bfs(int startVertex) {
- vector<bool> visited(numVertices, false);
- queue<int> queue;
- visited[startVertex] = true;
- queue.push(startVertex);
- while (!queue.empty()) {
- int currentVertex = queue.front();
- queue.pop();
- cout << currentVertex << " ";
- for (int neighbor : adjacencyList[currentVertex]) {
- if (!visited[neighbor]) {
- visited[neighbor] = true;
- queue.push(neighbor);
- }
- }
- }
- }
- };
复制代码 int main() {
// Initialize the graph and add edges
Graph graph(numVertices);
// Add edges using graph.addEdge(source, destination);
graph.bfs(0);
return 0;
}
3.**Java示例:**
- import java.util.ArrayList;
- import java.util.LinkedList;
- import java.util.List;
- import java.util.Queue;
- class Graph {
- private int numVertices;
- private List<List<Integer>> adjacencyList;
- public Graph(int vertices) {
- numVertices = vertices;
- adjacencyList = new ArrayList<>(numVertices);
- for (int i = 0; i < numVertices; i++) {
- adjacencyList.add(new ArrayList<>());
- }
- }
- public void addEdge(int source, int destination) {
- adjacencyList.get(source).add(destination);
- }
- public void bfs(int startVertex) {
- boolean[] visited = new boolean[numVertices];
- Queue<Integer> queue = new LinkedList<>();
- visited[startVertex] = true;
- queue.add(startVertex);
- while (!queue.isEmpty()) {
- int currentVertex = queue.poll();
- System.out.print(currentVertex + " ");
- for (int neighbor : adjacencyList.get(currentVertex)) {
- if (!visited[neighbor]) {
- visited[neighbor] = true;
- queue.add(neighbor);
- }
- }
- }
- }
- }
复制代码 public class Main {
public static void main(String[] args) {
// Initialize the graph and add edges
Graph graph = new Graph(numVertices);
// Add edges using graph.addEdge(source, destination);
graph.bfs(0);
}
}
4.**Python示例:**
- from collections import deque
- class Graph:
- def __init__(self, vertices):
- self.numVertices = vertices
- self.adjacencyList = [[] for _ in range(self.numVertices)]
- def addEdge(self, source, destination):
- self.adjacencyList[source].append(destination)
- def bfs(self, startVertex):
- visited = [False] * self.numVertices
- queue = deque()
- visited[startVertex] = True
- queue.append(startVertex)
- while queue:
- currentVertex = queue.popleft()
- print(currentVertex, end=" ")
- for neighbor in self.adjacencyList[currentVertex]:
- if not visited[neighbor]:
- visited[neighbor] = True
- queue.append(neighbor)
- # Initialize the graph and add edges
复制代码 graph = Graph(numVertices)
# Add edges using graph.addEdge(source, destination)
graph.bfs(0)
这些示例展示了使用不同编程语言实现广度优先搜索算法的方法。每个示例都使用类似的思路,通过使用队列来遍历图中的节点。您可以根据需要修改算法和输入图形。请注意,这只是广度优先搜索算法的简单示例,实际应用中可能会有更复杂的问题和算法。
|
|